.orderby()
The orderby method can be used to sort the result-set by one or more columns. Grouping and subtotals are calculated first and then ordering takes place.
orderby sorts the records in ascending order by default. Use desc to sort the records in a descending order.
.orderby(columnName: string)
var q = ml.query().from('Example/CampaignDonors'); q.select('State','Amount'); q.orderby('Amount.desc'); q.run(function (data, query)
<html> <head> <script type="text/javascript" src="/JS"></script> <script type="text/javascript"> ml.onload(function () { var q = ml.query().from('Example/CampaignDonors'); q.select('State','Amount'); q.orderby('Amount.desc'); q.run(function (data, query) { var dataDiv = document.getElementById("dataDiv"); var html = '<table><tr><th>State</th><th>Amount</th></tr>'; for(var i = 0; i < data.data.State.length; i++){ html += '<tr><td>' + data.data.State[i] + '</td>'; html += '<td>' + data.data.Amount[i] + '</td></tr>'; } html += '</table>'; dataDiv.innerHTML = html; }); }); </script> <style> table tr:nth-child(even) { background-color: #eee; } table tr:nth-child(odd) { background-color:#fff; } table th { background-color: rgb(63, 94, 144); color: white; padding: 5px; } table td { padding: 5px; } </style> </head> <body> <div id="dataDiv"></div> </body> </html>
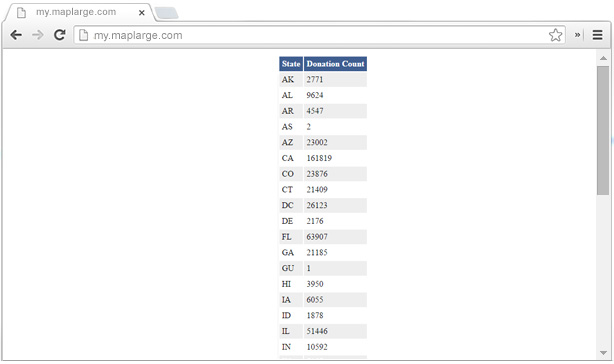
<html> <head> <script type="text/javascript" src="/JS"></script> <script type="text/javascript"> ml.onload(function () { var q = ml.query().from('Example/CampaignDonors'); // choose the columns to return q.select('State','Amount.sum'); // group the data q.groupby('State').orderby('Amount.sum.desc'); // execute the query and process the return with the callback q.run(function (data, query) { // get a reference to the element that will house our HTML table var dataDiv = document.getElementById("dataDiv"); // build the HTML table var html = '<table><tr><th>State</th><th>Donation Sum</th></tr>'; for(var i = 0; i < data.data.State.length; i++){ html += '<tr><td>' + data.data.State[i] + '</td>'; html += '<td>$' + ml.util.addCommas(data.data.Amount_Sum[i]) + '</td></tr>'; } html += '</table>'; dataDiv.innerHTML = html; }); }); </script> <style> table tr:nth-child(even) { background-color: rgb(231, 231, 231) } table tr:nth-child(odd) { background-color:#fff; } table th { background-color: rgb(86, 110, 140); color: white; padding: 5px; } table td { padding: 5px; } </style> </head> <body> <div id="dataDiv"></div> </body> </html>
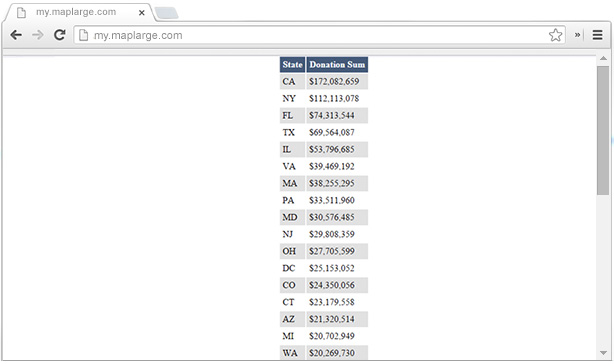
<html> <head> <script type="text/javascript" src="/JS"></script> <script type="text/javascript"> ml.onload(function () { var q = ml.query().from('Example/CampaignDonors'); // choose the columns to return q.select('State','Amount.avg'); // group the data q.groupby('State').orderby('Amount.avg.desc').take('10'); // execute the query and process the return with the callback q.run(function (data, query) { // get a reference to the element that will house our HTML table var dataDiv = document.getElementById("dataDiv"); // build the HTML table var html = '<table><tr><th>State</th><th>Donation Avg</th></tr>'; for(var i = 0; i < data.data.State.length; i++){ html += '<tr><td>' + data.data.State[i] + '</td>'; html += '<td>$' + ml.util.addCommas(data.data.Amount_Avg[i]) + '</td></tr>'; } html += '</table>'; dataDiv.innerHTML = html; }); }); </script> <style> table tr:nth-child(even) { background-color: rgb(231, 231, 231) } table tr:nth-child(odd) { background-color:#fff; } table th { background-color: rgb(86, 110, 140); color: white; padding: 5px; } table td { padding: 5px; } </style> </head> <body> <div id="dataDiv"></div> </body> </html>
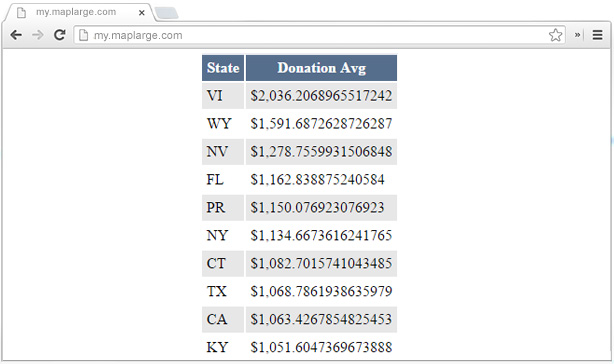