JavaScript API Integration
Google Maps API code is drop-in compatible with the MapLarge platform. There are two methods for integrating your map.
Method 1: Create a Google Map with MapLarge Map Constructor
Add a MapLarge wrapper at the beginning of your javascript where the google map is instantiated and configured.
<!DOCTYPE html> <html> <head> <title>Simple Map</title> <script type="text/javascript" src="//e.maplarge.com/JS"></script> <script type="text/javascript" src="//maps.googleapis.com/maps/api/js?libraries=visualization,drawing&sensor=false"></script> <script type="text/javascript"> var map; ml.onload( function () { // add a hotel layer using ML as the icon text var layers = [{ onClick: "template", query: { select: { type: "geo.dot" }, where: [], table: { name: "hms/hotels" } }, style: { method: "rules", rules: [ { style: { fillColor: "Blue", size: 24, text: { x: 4, y: 7, text: "ML" } }, where: [ { col: "hms/hotels.HotelName", test: "Contains", value: "hilton" } ] } ], colorTransform: { alpha: .74 } }, onHover: "template", hoverTemplate: "{HotelName}", hoverFieldsCommaDel: "HotelName", clickTemplate: "{HotelName}", visible: true }]; //create a map zoomed in on Atlanta, GA (34,-84) //the second parameter is a Object literal var div = document.getElementById('map-canvas'); var mlmap = new ml.map( div, { layers: layers, lat: 33.7514, lng: -84.3378, z: 9, api:"GOOGLE" } ); map = mlmap.getInternalMap(); //======================== var myLatlng = new google.maps.LatLng(34,-84); var myLatlng1 = new google.maps.LatLng(34.11,-84.15); var myLatlng2 = new google.maps.LatLng(34.15,-84.12); var myLatlng3 = new google.maps.LatLng(34.18,-84.09); var myLatlng4 = new google.maps.LatLng(34.20,-84.04); var trafficLayer = new google.maps.TrafficLayer(); trafficLayer.setMap(map); var marker = new google.maps.Marker({ position: myLatlng, map: map, title: 'Hello World!' }); var marker1 = new google.maps.Marker({ position: myLatlng1, map: map, title: 'Hello World!' }); var marke2r = new google.maps.Marker({ position: myLatlng2, map: map, title: 'Hello World!' }); var marker3 = new google.maps.Marker({ position: myLatlng3, map: map, title: 'Hello World!' }); var marker4 = new google.maps.Marker({ position: myLatlng4, map: map, title: 'Hello World!' }); }); </script> <meta name="viewport" content="initial-scale=1.0, user-scalable=no"> <meta charset="utf-8"> <style> html, body, #map-canvas { height: 100%; margin: 0px; padding: 0px } </style> </head> <body> <div id="map-canvas"></div> </body> </html>
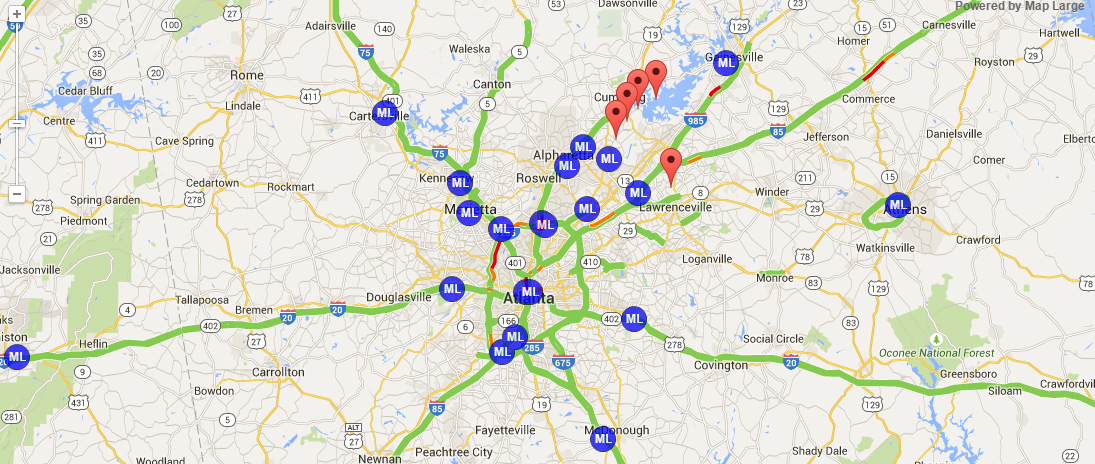
Advantages
Seamless API Changing | Allows seamless switching to other APIs like Leaflet, ESRI and OpenGeo for code that is coded against the MapLarge map wrapper object. |
Raw Google Map Access | Also gives the flexibility to grab the internal google map for google specific calls. |
Disadvantages
Map Constructor Change | Requires replacing existing google maps constructor and then calling mlmap.getInternalMap() to get a reference to the internal Google Map. |
Method 2: Google Map Directly and Pass it to MapLarge Map Constructor
Create a MapLarge map instantiated using the existing Google Map API code.
<!DOCTYPE html> <html> <head> <title>Simple Map</title> <script type="text/javascript" src="//e.maplarge.com/JS"></script> <script type="text/javascript" src="//maps.googleapis.com/maps/api/js?libraries=visualization,drawing&sensor=false"></script> <script type="text/javascript"> ml.onload(function() { var startZoom = 10; var startLat = 38.886349760669056; var startLng = -77.00554275512695; var mapOptions = { center: new google.maps.LatLng(startLat, startLng), zoom: startZoom, mapTypeId: google.maps.MapTypeId.TERRAIN }; var googMap = new google.maps.Map(document.getElementById("map-canvas"), mapOptions); var marker = new google.maps.Marker({ position: mapOptions.center, map: googMap, title: 'Hello World!' }); var mlmap = new ml.map(googMap); });</script> <meta name="viewport" content="initial-scale=1.0, user-scalable=no"> <meta charset="utf-8"> <style> html, body, #map-canvas { height: 100%; margin: 0px; padding: 0px } </style> </head> <body> <div id="map-canvas"></div> </body> </html>
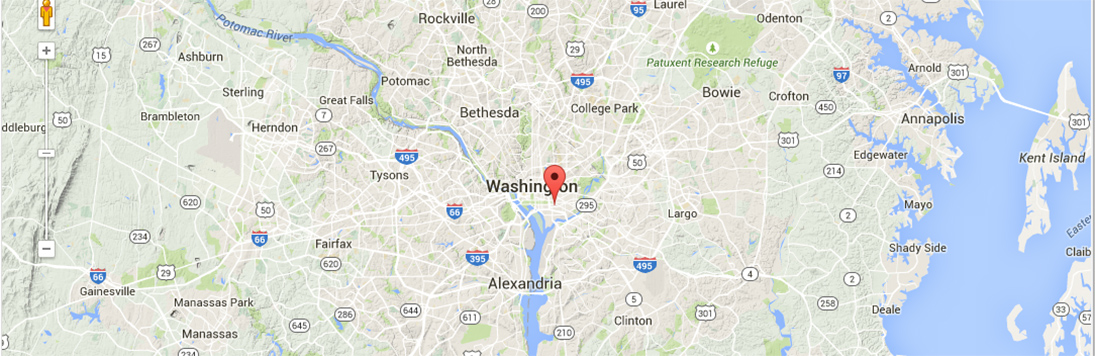
Advantages
Low Effort | Allows adding a MapLarge Layer with zero changes to existing code. |
Disadvantages
Not Portable to Other API’s | Code that does not use the MapLarge map wrapper object does not allow fluid switching between different client side API’s like Leaflet and ESRI. |