.groupby(columnName.rules)
groupby rules provides the ability to group a column by a set of rules. This feature is currently only implemented on numeric column types.
The groupby rules are composed of a pipe ( | ) separated list of min/max pairs with the option to use the keyword “catch” as a catch all (* in SQL).
Rules do not have to be in numeric order but catch is required to group non-matching rows.
.groupby(columnName.rules.min1/max1|min2/max2|min3/max3)
var q = ml.query().from('maplarge/Donors'); q.select('Amount.sum','Amount.count','Amount.key','Amount'); q.groupby('Amount.rules.0/1000|1000/10000|10000/100000|100000/1000000|catch'); q.run(function (data, query); console.log(data); });
<html> <head> <script type="text/javascript" src="/JS"></script> <script type="text/javascript"> ml.onload(function () { var q = ml.query().from('maplarge/Donors'); // choose the columns to return q.select('Amount.sum','Amount.count','Amount.key','Amount'); // group the data q.groupby('Amount.rules.0/1000|1000/10000|10000/100000|100000/1000000|catch'); // execute the query and process the return with the callback q.run(function (data, query) { // get a reference to the element that will house our HTML table var dataDiv = document.getElementById("dataDiv"); // build the HTML table var html = '<table><tr><th></th><th>1</th><th>2</th><th>3</th><th>4</th><th>5</th></tr>'; html += '<tr><td>Donation Count</td>'; for(var i = 0; i < data.data.Amount_Count.length; i++){ html += '<td>' + ml.util.addCommas(data.data.Amount_Count[i]) + '</td>'; } html += '</tr>'; html += '<tr><td>Donation Sum</td>'; for(var i = 0; i < data.data.Amount_Sum.length; i++){ html += '<td>$ ' + ml.util.addCommas(data.data.Amount_Sum[i]) + '</td>'; } html += '</tr>'; html += '</table>'; dataDiv.innerHTML = html; }); }); </script> <style> table tr:nth-child(even) { background-color: #eee; } table tr:nth-child(odd) { background-color:#fff; } table th { background-color: rgb(82, 112, 158); color: white; } table td { text-align:right; padding: 5px; } table tr td:nth-child(1) { text-align:left; } </style> </head> <body> <div id="dataDiv"></div> </body> </html>
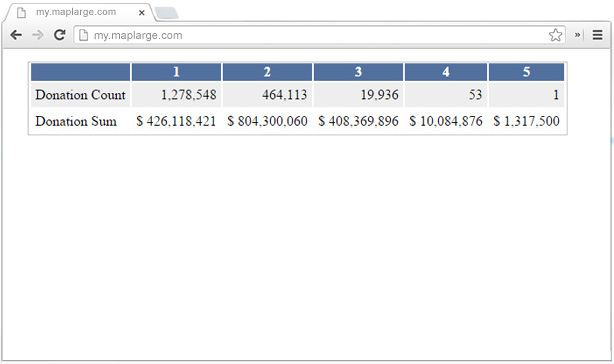